Problem Description
Tom is a commander, his task is destroying his enemy’s transportation system. Let’s represent his enemy’s transportation system as a simple directed graph G with n nodes and m edges. Each node is a city and each directed edge is a directed road. Each edge from node u to node v is associated with two values D and B, D is the cost to destroy/remove such edge, B is the cost to build an undirected edge between u and v. His enemy can deliver supplies from city u to city v if and only if there is a directed path from u to v. At first they can deliver supplies from any city to any other cities. So the graph is a strongly-connected graph. He will choose a non-empty proper subset of cities, let’s denote this set as S. Let’s denote the complement set of S as T. He will command his soldiers to destroy all the edges (u, v) that u belongs to set S and v belongs to set T. To destroy an edge, he must pay the related cost D. The total cost he will pay is X. You can use this formula to calculate X:
After that, all the edges from S to T are destroyed. In order to deliver huge number of supplies from S to T, his enemy will change all the remained directed edges (u, v) that u belongs to set T and v belongs to set S into undirected edges. (Surely, those edges exist because the original graph is strongly-connected) To change an edge, they must remove the original directed edge at first, whose cost is D, then they have to build a new undirected edge, whose cost is B. The total cost they will pay is Y. You can use this formula to calculate Y:
At last, if Y>=X, Tom will achieve his goal. But Tom is so lazy that he is unwilling to take a cup of time to choose a set S to make Y>=X, he hope to choose set S randomly! So he asks you if there is a set S, such that Y<X. If such set exists, he will feel unhappy, because he must choose set S carefully, otherwise he will become very happy.

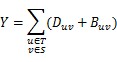
Input
There are multiply test cases. The first line contains an integer T(T<=200), indicates the number of cases. For each test case, the first line has two numbers n and m. Next m lines describe each edge. Each line has four numbers u, v, D, B. (2=<n<=200, 2=<m<=5000, 1=<u, v<=n, 0=<D, B<=100000) The meaning of all characters are described above. It is guaranteed that the input graph is strongly-connected.
Output
For each case, output "Case #X: " first, X is the case number starting from 1.If such set doesn’t exist, print “happy”, else print “unhappy”.
Sample Input
2
3 3
1 2 2 2
2 3 2 2
3 1 2 2
3 3
1 2 10 2
2 3 2 2
3 1 2 2
Sample Output
Case #1: happy
Case #2: unhappy
Sample Output
In first sample, for any set S, X=2, Y=4.
In second sample. S= {1}, T= {2, 3}, X=10, Y=4.
题意
给你N个点M条边强连通的有向简单图,D代表删掉这个边的花费,D+B代表重建为双向边的花费,让你选择一个集合S,其余的点在T集合,X为u在S集合v在T集合的所有边的D之和,Y为u在T集合v在S集合的所有边的D+B之和,求是否存在一个集合S,使得X>Y,若存在输出unhappy,否则输出happy
题解
无源汇上下界网络流,下界D,上界D+B,判断是否存在可行流
若存在,则说明对于任意集合S,流出的流量=流入的流量,X<=流出的流量<=Y
建图每条边建为自由流(u,v,B)
对于每个点,设M为总流入-总流出
若M>0,则建(S,i,M)说明i需要多流出M
若M<0,则建(i,T,M)说明i需要多流入M
最后判断与S连的边是否全满流
代码
1 #include2 using namespace std; 3 4 const int maxn=1e5+5; 5 const int maxm=2e5+5; 6 const int INF=0x3f3f3f3f; 7 8 int TO[maxm],CAP[maxm],NEXT[maxm],tote; 9 int FIR[maxn],gap[maxn],cur[maxn],d[maxn],q[400000];10 int n,m,S,T;11 12 void add(int u,int v,int cap)13 {14 TO[tote]=v;15 CAP[tote]=cap;16 NEXT[tote]=FIR[u];17 FIR[u]=tote++;18 19 TO[tote]=u;20 CAP[tote]=0;21 NEXT[tote]=FIR[v];22 FIR[v]=tote++;23 }24 void bfs()25 {26 memset(gap,0,sizeof gap);27 memset(d,0,sizeof d);28 ++gap[d[T]=1];29 for(int i=1;i<=n;++i)cur[i]=FIR[i];30 int head=1,tail=1;31 q[1]=T;32 while(head<=tail)33 {34 int u=q[head++];35 for(int v=FIR[u];v!=-1;v=NEXT[v])36 if(!d[TO[v]])37 ++gap[d[TO[v]]=d[u]+1],q[++tail]=TO[v];38 }39 }40 int dfs(int u,int fl)41 {42 if(u==T)return fl;43 int flow=0;44 for(int &v=cur[u];v!=-1;v=NEXT[v])45 if(CAP[v]&&d[u]==d[TO[v]]+1)46 {47 int Min=dfs(TO[v],min(fl,CAP[v]));48 flow+=Min,fl-=Min,CAP[v]-=Min,CAP[v^1]+=Min;49 if(!fl)return flow;50 }51 if(!(--gap[d[u]]))d[S]=n+1;52 ++gap[++d[u]],cur[u]=FIR[u];53 return flow;54 }55 int ISAP()56 {57 bfs();58 int ret=0;59 while(d[S]<=n)ret+=dfs(S,INF);60 return ret;61 }62 void init()63 {64 tote=0;65 memset(FIR,-1,sizeof FIR);66 }67 int in[maxn];68 int main()69 {70 int t;71 scanf("%d",&t);72 for(int ca=1;ca<=t;ca++)73 {74 init();75 memset(in,0,sizeof in);76 scanf("%d%d",&n,&m);77 for(int i=0,u,v,d,b;i 0)88 {89 add(S,i,in[i]);90 sum+=in[i];91 }92 else if(in[i]<0)93 add(i,T,-in[i]);94 printf("Case #%d: %s\n",ca,ISAP()==sum?"happy":"unhappy");95 }96 return 0;97 }